Maintaining good mental health is extremely important.
Most of us lead very busy lives, and it is really important to take our stress levels under control.
That is why we have come up with an excellent project for you. In this post, we will walk you through a mental health tracker project with Python.
It is pretty simple and straightforward. So let’s get started.
This mental health tracker project is intended to assist users in tracking their mood and stress levels on a daily basis. Also, any user can save that data for later retrieval.
You or your intended users may utilize this project to get insight into how the mood changes over time. Aldo, you can take proactive efforts to improve it.
Use of Python
The project is written in Python and includes the CSV library. Also, we make use of a basic command-line interface for inputting and displaying data. It’s a simple project that may be further developed to handle additional sorts of data.
These could include saving triggers, thoughts, and actions of a person.
This tutorial will guide you through the code as well as demonstrate how to set up and run the project. It will also examine potential project changes that may increase its functioning.
Prerequisites
You must have Python installed on your computer to complete this project. Python may be downloaded from the official Python website (https://www.python.org/downloads/). You should also ensure that you have the right Python version for the code you intend to run.
The project also makes use of the CSV library, which is included with Python. Thus, you don’t need to download extra libraries. You should always note that to avoid compatibility difficulties, you have the most recent version of the libraries.
Tutorial
Create a new file in your VSCode called “mental_health_tracker.py”
We begin by importing the CSV library. It will be used to read and write data to CSV files.
import csv
Following that, we define three functions:
- The function “create tracker(filename)” will create a new CSV file. It comes with a specified filename and writes the headers for the data. We will be collecting data, mood, and stress levels.
- The “add_entry(filename)” function allows the user to enter their mood and stress level for a specific day. It is done before saving that data to the CSV file indicated by the filename.
- function “view_entries(filename)” Read and print the data from the CSV file.
def create_tracker(filename):
# Create the CSV file and write the headers
with open(filename, 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['date', 'mood', 'stress_level'])
def add_entry(filename):
date = input("Enter date (YYYY-MM-DD): ")
mood = input("Enter your mood (1-5): ")
stress_level = input("Enter your stress level (1-5): ")
# Append the data to the CSV file
with open(filename, 'a', newline='') as file:
writer = csv.writer(file)
writer.writerow([date, mood, stress_level])
print("Data saved successfully!")
def view_entries(filename):
# Read the data from the CSV file and print it out
with open(filename, 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
If __name__ == ‘__main__’ in the main function. We define the filename for the CSV file that will be used by the application. And, we create a tracker method to create the file if it does not already exist.
Then we create a simple loop that invites the user to perform an action. For example; we will be adding an entry, viewing existing entries, or exiting the application. Hence, we can run the appropriate function based on their input.
if __name__ == '__main__':
filename = 'tracker.csv'
create_tracker(filename)
while True:
action = input("Enter 'a' to add entry, 'v' to view entries, 'q'
to quit: ")
if action == 'a':
add_entry(filename)
elif action == 'v':
view_entries(filename)
elif action == 'q':
break
else:
print("Invalid action, Please try again!")
Finally, it does a basic validation of the user’s action and prints an error message if it is not ‘a’,’v’,’q’.
This is only a basic example, but you can easily add more functionality as needed.
You may also want to consider checking the user’s data. For example, the date should be in the right format, and the mood and stress level should be between 1 and 5.
Running the Project
Type python mental_health_tracker.py
to run the script.
Follow the prompt and type the required information.
Also, you may see the data by rerunning the script and selecting the display option.
Possible Improvements
- Data validation can be included. For example, you can ask the user to validate the date format, mood, and stress level as it is being added.
- You can incorporate several data kinds, including behaviors, ideas, and triggers.
- You can make the data visually appealing with a tool like Matplotlib or Seaborn. This might make it simpler to see patterns and trends over time.
- You can make the project more user-friendly. You could use a graphical user interface (GUI) instead of a command-line interface (CLI).
- You can give the data extra features like search and filtering.
Conclusion
We’ve covered the fundamentals of creating a mental health tracker in Python using the CSV module. We believe that tracking our mental health gives us insight into how it changes over time.
Hence, we can take farsighted measures to enhance it. In order to make the project more functional, we’ve also spoken about prospective improvements.
This project is a straightforward yet efficient approach to measuring our mental health, which is a vital step in maintaining excellent overall health. Thank you for reading this post. We sincerely hope it will be helpful to you as you work to achieve better mental health.
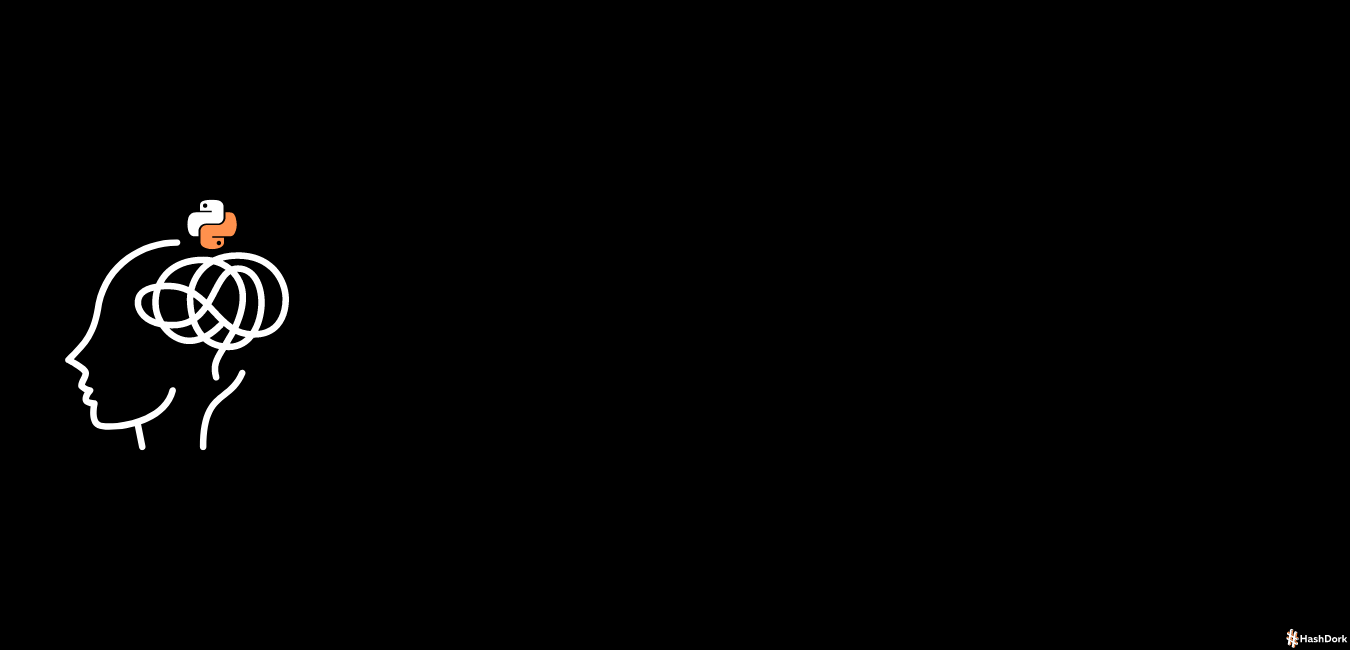
Leave a Reply