Table of Contents[Hide][Show]
- 1. What is Python scripting, and how does it differ from Python programming?
- 2. How does Python’s garbage collection work?
- 3. Explain the difference between a list and a tuple
- 4. What are list comprehensions and give an example of their usage?
- 5. Describe the difference between deepcopy and copy?
- 6. How is multithreading achieved in Python and how does it differ from multiprocessing?
- 7. What are decorators and how are they used in Python?
- 8. Explain the differences between *args and **kwargs?
- 9. How would you ensure a function can only be called once using decorators?
- 10. How does inheritance work in Python?
- 11. What is method overloading and overriding?
- 12. Describe the concept of polymorphism with an example.
- 13. Explain the difference between instance, class, and static methods.
- 14. Describe how a Python set works internally.
- 15. How is a dictionary implemented in Python?
- 16. Explain the benefits of using named tuples.
- 17. How does the try-except block work?
- 18. What is the difference between raise and assert statements?
- 19. How do you read and write data from a binary file in Python?
- 20. Explain the with statement and its advantages when working with file I/O.
- 21. How would you create a singleton module in Python?
- 22. Name a few ways to optimize memory usage in a Python script.
- 23. How would you extract all email addresses from a given string using regex?
- 24. Explain the Factory design pattern and its application in Python
- 25. What is the difference between an iterator and a generator?
- 26. How does the @property decorator work?
- 27. How would you create a basic REST API in Python?
- 28. Describe how to use the requests library to make an HTTP POST request.
- 29. How would you connect to a PostgreSQL database using Python?
- 30. What’s the role of ORMs in Python and name a popular one?
- 31. How would you profile a Python script?
- 32. Explain the GIL (Global Interpreter Lock) in CPython
- 33. Explain Python’s async/await. How is it different from traditional threading?
- 34. Describe how you would use Python’s concurrent.futures.
- 35. Compare Django and Flask in terms of use case and scalability.
- Conclusion
In a time when technology exists in every aspect of our lives, Python scripting emerges as a key component of the enormous and intricate IT infrastructure, ushering in a paradigm of ease of use and usefulness.
Python’s strength resides not only in its syntactical simplicity and readability but also in its adaptability, which allows it to bridge the gap between low-risk, beginner-level scripting and high-stakes, enterprise-level software development with ease.
Python’s wide libraries and frameworks pave the way for a fluid, imaginative technical adventure, whether it be in the areas of data analysis, web development, artificial intelligence, or network servers.
In addition to being a tool for problem-solving, Python also fosters an atmosphere where innovation is not only embraced but also naturally incorporated thanks to its enormous libraries and frameworks, such as Django for web development or Pandas for data analysis.
In a world where data is king, Python provides powerful tools for manipulating, analyzing, and visualizing data, resulting in actionable insights and guiding strategic choices.
Python is not simply a programming language; it is also a thriving community, a hub where developers, data scientists, and tech enthusiasts get together to invent, create, and take the IT industry to the next level.
Python developers are sought after by businesses of all sizes, from fledgling startups to well-established organizations, as catalysts for innovation, process improvement, and improved customer service.
Additionally, it’s open-source nature fosters a culture of shared learning and collaborative growth, guaranteeing that it will continue to advance with the rapidly changing technological world.
Learning Python in 2023 is an investment in a language that promises to remain current, flexible, and essential for managing the ebbs and flows of technology.
It gives access to the fields of machine learning, data analytics, cybersecurity, and more, all of which are crucial to shaping the digital era.
Therefore, we have compiled a list of the best Python scripting interview questions for you, which will enable you to shine as a developer and ace the interview.
1. What is Python scripting, and how does it differ from Python programming?
Python is known for its adaptability and provides both scripting and programming skills, each suited to particular jobs and goals.
Python scripting is fundamentally the process of writing shorter, more efficient scripts that are intended to manage files, automate repetitive processes, or quickly prototype ideas.
These scripts, which are frequently stand-alone, efficiently carry out a list of actions in order.
Python programming, on the other hand, goes further, emphasizing the creation of bigger, more intricate programs with structured code using libraries, frameworks, and best practices.
While they both come from the same language, scripting simplifies and automates while programming creates and invents. This difference can be seen in the scope and goals of each discipline.
2. How does Python’s garbage collection work?
A key element in ensuring effective memory management is Python’s garbage collection system.
It tirelessly works in the background to protect system resources from being overrun by memory leaks. This automated approach is based mostly on the reference counting method, where each object keeps track of how many other objects are referencing it.
This object becomes a candidate for memory reclamation when this count falls to 0, which indicates the item is no longer required.
In addition, Python uses a cyclic garbage collector, which the simple reference count approach could miss, to find and clear up reference cycles.
Thus, the reference counting and cyclic garbage collection dual-layered strategy provides a careful and effective use of memory, strengthening Python’s performance, especially in memory-intensive applications.
A simple code sample showing how to interface with Python’s garbage collection system is provided below:
Two objects are generated in this excerpt and cross-referenced to establish a cycle. The garbage collector is then manually triggered using gc.collect(), showing how programmers can engage with Python’s memory management mechanism as necessary.
3. Explain the difference between a list and a tuple
Lists and tuples are effective containers for data in the Python world, but they have different properties that meet different programming purposes.
A list, denoted by square brackets, enables flexibility by allowing for the changing and dynamic resizing of its components.
A tuple enclosed in parenthesis, on the other hand, is immutable and maintains its initial state while the function is being executed.
Tuples give a solid, immutable sequence whereas lists offer flexibility, allowing for a variety of uses in data processing and modification.
Here is a little Python code sample showing how to utilize both lists and tuples:
4. What are list comprehensions and give an example of their usage?
List comprehensions are an efficient and expressive way to create lists in Python that combine the power of conditional logic and loops into a single, understandable line of code.
They provide a simplified syntax to convert our intents into a list, combining iteration and conditionality into a single, refined structure.
List comprehensions essentially give programmers the ability to create lists by executing operations on each member and maybe filtering them depending on certain criteria, all while keeping a tidy codebase.
This expressive feature combines efficiency with clarity in Python programming by improving readability while also possibly providing computational gains in some circumstances.
An illustration of a Python list comprehension is shown below:
5. Describe the difference between deepcopy and copy?
The depth and integrity of the duplicated objects determine the difference between deepcopy
and copy
in Python.
By creating a new item while keeping references to the original nested objects, a copy
creates a shallow replica that weaves their fates together in a web of interdependence.
Deepcopy
creates a totally autonomous clone by recursively copying the original object and all of its hierarchical components, cutting all connections and maintaining autonomy in changes.
Therefore, depending on the required level of object independence, deepcopy
assures a comprehensive reproduction whereas copy just gives a surface-level duplication.
Here is some code to show how copy
and deepcopy
vary from one another:
6. How is multithreading achieved in Python and how does it differ from multiprocessing?
Python’s multiprocessing and multithreading both address concurrent execution, but using different paradigms.
Using many threads inside a single process, multithreading enables concurrent task execution within a shared memory space.
However, genuine parallel thread execution might be difficult to achieve because of Python’s Global Interpreter Lock (GIL).
On the other hand, multiprocessing makes use of several processes, each with a separate Python interpreter and memory space, ensuring true parallelism.
For I/O-bound activities, multithreading is more lightweight and practical, but multiprocessing excels in CPU-bound situations where real parallel execution is crucial.
Here is a brief code sample that contrasts multiprocessing vs multithreading:
7. What are decorators and how are they used in Python?
In Python, decorators elegantly combine usefulness and simplicity while subtly augmenting or changing functions.
Think of decorators as a veil that beautifully envelops a function, adding to its capabilities without changing its essential nature.
These entities, denoted by the symbol @
, accept a function as input and output a whole new function, offering a seamless means of modifying function behavior.
Decorators impart a wide range of features, from logging to access control, enhancing the code with new layers while maintaining a clear, understandable syntax.
Here is a simple Python code example showing how decorators are used:
8. Explain the differences between *args and **kwargs?
Python’s flexible parameters *args
and **kwargs
allow functions to properly take a range of arguments.
A function can accept any number of positional arguments using the *args
parameter, which groups them into a tuple.
In contrast, a function can accept any number of keyword arguments using the **kwargs
parameter, which groups them into a dictionary.
Both act as channels for dynamism and flexibility in function construction and calling, **kwargs
offering a structured method for handling an arbitrary amount of keyword inputs while *args
gracefully handles undefined positional inputs.
Together, they improve the flexibility and durability of Python functions by skillfully and clearly handling a wide range of application scenarios.
An example of Python code that uses *args
and **kwargs
is provided below:
9. How would you ensure a function can only be called once using decorators?
Python decorators are adept at combining usefulness with elegance, which is needed to ensure a function’s singularity in execution.
It is possible to design a decorator to enclose a function and keep track of this information inside by keeping an internal state.
The encapsulated function is called once, and executed, and the decorator records the call. Subsequent calls are blocked, protecting the function from repeated executions by ensuring it is not disturbed.
With the help of this application of decorators, function calls can be controlled in a subtle yet effective way, guaranteeing uniqueness in a way that is both beautiful and unobtrusive.
Here is a code sample to show how decorators can be used to limit the number of times a function can be called:
10. How does inheritance work in Python?
Python’s inheritance system creates a web of hierarchical links between classes, allowing characteristics and functions from a parent class to be shared with its offspring.
It manages a lineage that allows derived (child) classes to inherit, replace, or add functionality from their base (parent) classes, promoting code reuse and a logical, hierarchical design.
The child class can introduce its unique features and behaviors in addition to absorbing capabilities from its parent, creating a strong, multi-layered object model.
In this approach, inheritance skillfully distributes functionality throughout the class hierarchy’s arteries, creating a unified, well-organized object-oriented architecture.
The following simplified Python code demonstrates inheritance:
11. What is method overloading and overriding?
The two cornerstones of object-oriented programming, method overloading and method overriding, enable developers to use the same method name for several purposes.
A single method can accommodate a variety of data types and argument counts by having many signatures thanks to method overloading.
On the other hand, method overriding allows a subclass to add its own special implementation to a method that is already defined in its parent class, guaranteeing that the child’s version is called.
Together, these strategies improve adaptability by enabling method behaviors that depend on context and the particular requirements of the application.
Here is a sample of code that exemplifies both concepts:
12. Describe the concept of polymorphism with an example.
Polymorphism is the practice of using a single interface for various data types.
This idea ensures adaptability and scalability in design by giving methods the freedom to process objects in multiple ways depending on their intrinsic type or class.
In essence, polymorphism enables unified interactions while keeping distinct behaviors by allowing objects of different classes to be considered as instances of the same class through inheritance.
This dynamic feature encourages code simplicity by allowing a single function or operator to interact with a variety of object kinds without any problems.
Here is a clear code sample that demonstrates polymorphism:
13. Explain the difference between instance, class, and static methods.
Instance, class, and static methods all have their own distinct ways of interacting with object and class data in Python.
The most prevalent kind, instance methods, act on class instance data and take as input an instance of the class, typically called self.
The class itself (often referred to as cls) is accepted as an argument by class methods, which are denoted with @classmethod, and they manipulate class-level data.
Static methods, denoted by the hash symbol @staticmethod, don’t affect class or instance states since they are freestanding functions contained within the class and don’t take self or cls as a first parameter.
Because each method type provides different access and utility, object-oriented architectures are flexible and precise.
As an example of one of these method types in code:
14. Describe how a Python set works internally.
An internal data structure called a hashtable is used by a Python set, which is an unordered collection of distinct components, to perform powerful and effective operations.
Python uses a hash function to quickly manage and retrieve data when an element is added to a set, turning the element into a hash value that then defines its location in memory.
By facilitating quick membership checks and removing duplicate entries, this technique makes sure that every element in a set is unique and easily accessible.
Therefore, the inherent architecture of sets tends to optimize operations like unions, crossings, and differences, resulting in a small, effective data structure.
Here is a piece of code that shows how to interact with a Python set simply:
15. How is a dictionary implemented in Python?
A hashtable serves as the foundation of a dictionary in Python and allows for quick data retrieval and manipulation. Dictionaries are dynamic, unordered collections of key-value pairs.
Python uses a hash function to compute the key’s hash when a key-value pair is issued, locating the location of the value’s storage address in memory.
As the hash function immediately points the interpreter to the memory address, this design offers quick access to data based on keys and is astonishingly efficient in retrieval, insertion, and deletion operations.
Devs can manage data easily and effectively because of the enticing combination of speed and flexibility provided by Python dictionaries.
Listed below is a code sample showing how to use a Python dictionary:
16. Explain the benefits of using named tuples.
The use of named tuples in Python skillfully combines the expressiveness of classes with the simplicity of tuples, resulting in a small, self-explanatory data structure.
The traditional tuple is extended by named tuples, which keep the immutability and memory efficiency of tuples while adding named fields to improve code readability and self-description.
Named tuples promote clear, understandable, and performant code by establishing straightforward, lightweight objects without any methods, improving both the developer experience and computational performance.
As a result, named tuples develop into a powerful tool that improves data structure and readability without compromising speed.
A code sample illustrating the use of named tuples is shown below:
17. How does the try-except block work?
The try-except block acts as a sentinel in Python expressive syntax, vigilantly guarding against runtime irregularities and maintaining the execution’s smooth flow despite potential problems.
When a try block encounters an error, the control is automatically transferred to the appropriate except block, where the problem is fixed by reporting, fixing, or maybe rethrowing the exception.
By handling exceptions in a purposeful, controlled way, this system not only protects against disruptive crashes but also improves user experience and data integrity.
As a result, the try-except block skillfully blends error management with program execution, guaranteeing application robustness and stability.
Here is a little sample of code that uses the try-except block:
18. What is the difference between raise and assert statements?
The raise and assert statements in Python’s error handling represent two separate but related expressions of exception management.
The raise
statement gives the programmer explicit control over error messages and flow by allowing them to explicitly cause specified exceptions.
Assert
, on the other hand, acts as a debugging tool by automatically generating an AssertionError
if its corresponding condition is not satisfied, guaranteeing that the program performs as intended during development.
Assert
simply checks conditions, improving debugging and validation, whereas raise enables wider, more explicit control. Both raise and assert permit controlled exception production.
Here is some sample code showing how to use raise
and assert
:
19. How do you read and write data from a binary file in Python?
Using the built-in open function with a binary mode specifier, interfacing with binary files in Python entails a balance of accuracy and simplicity.
Using the rb
or wb
modes when opening a binary file will ensure that the data is treated in its unencoded, raw form when reading or writing binary data.
By using these modes, Python simplifies the management of non-text data, such as pictures or executable files, enabling programmers to handle and analyze binary data precisely and easily.
Therefore, binary file operations in Python open the door to a broad range of applications, including data serialization, image processing, and binary analysis, to mention a few.
Using a binary file, this example of code shows how to read and write data:
20. Explain the with
statement and its advantages when working with file I/O.
Python’s with statement, which is frequently used with file I/O, elegantly makes sure that resources are handled effectively thanks to the idea of context management.
When dealing with files, with
statement immediately closes the file after use, even if an exception occurs while the action is being performed, protecting against resource leaks and guaranteeing a clean termination.
By eliminating boilerplate code, this syntactic sugar improves code readability. It also increases dependability and simplicity by integrating resource management and exception handling.
As a result, the with statement becomes essential for ensuring that your file operations are reliable and cleanly contained, protecting against unforeseen problems and improving code clarity.
Here is an example of code that uses the with
statement in file operations:
21. How would you create a singleton module in Python?
A combination of class methods and internal checks are used to create a singleton module in Python, a design pattern that only permits the creation of a single instance of a class.
By maintaining track of its own instance and providing a method to generate or return it, a class follows this pattern to make sure that subsequent instantiations replicate the first instance.
With a single point of control, unified access to resources, and protection against competing manipulations, singleton assures a single point of control.
As a result, it develops into an effective tool for encapsulating shared resources, guaranteeing consistent access and modification across the program.
Here is a little Python code sample demonstrating a singleton class:
22. Name a few ways to optimize memory usage in a Python script.
Python script memory consumption optimization frequently entails a careful balancing act between data structure choice, algorithm improvement, and resource management.
When working with huge datasets, for example, using generators rather than lists can significantly minimize memory use by lazy assessing the items on the fly rather than keeping them in memory.
Further reducing memory use is possible by handling numerical data with array data structures rather than lists and by sparingly using __slots__
in-class declarations to control the formation of dynamic attributes.
Thus, by balancing performance and resource use, you can ensure that Python programs are not only effective but also thoughtful in how much memory they use.
Here is a short example of code that uses a generator to reduce the amount of memory used:
23. How would you extract all email addresses from a given string using regex?
Regular expressions (regex) in Python combine accuracy and versatility to extract email addresses from a string, allowing the developer to deftly filter through textual material and identify desirable patterns.
To establish the structure of an email address, one creates a regex pattern using the re-module. Then, you can use findall
to get all occurrences from the target string.
This method expertly navigates the textual maze to obtain all hidden email addresses, which not only speeds up the extraction process but also assures correctness.
Regex can be skillfully used to effectively extract certain data from strings, increasing Python scripts’ data processing and analysis.
Here is a piece of code that uses regex to extract emails:
24. Explain the Factory design pattern and its application in Python
The fundamental tenet of object-oriented programming, the factory design pattern, is the creation of objects without identifying the precise class of the objects to be generated.
The Factory pattern can be elegantly implemented in Python by creating a method that returns instances of several classes depending on method inputs or configurations.
This procedure, which is sometimes referred to as a “Factory,” acts as a hub for weaving several class instances, guaranteeing that objects are created without the caller having to manually instantiate classes.
Thus, the Factory pattern maintains a decoupled, scalable architecture while improving code modularity and cohesiveness. It also offers a simplified technique to build objects.
25. What is the difference between an iterator and a generator?
It is clear from Python’s iterators and generators that both constructions make it possible to loop through values, however, there are subtle differences in how they are implemented and used.
A generator, which is frequently identified by its use of yield, automatically maintains its state and is implemented with a function, providing a concise and memory-efficient way to produce values on the fly.
An iterator, which is typically implemented as a class, uses methods like __iter__
and __next__
to manage its iteration state and produce values.
As a result, each has its own merits based on the particular use case, with iterators offering a thorough, object-oriented way to traverse over data while generators offer a lightweight, lazy evaluation technique.
Both techniques add to the developer’s arsenal and make it possible to explore data quickly and effectively in a variety of situations.
Here is a piece of code of an iterator and a generator in Python:
26. How does the @property
decorator work?
The ‘@property’ decorator in Python plays a lovely melody that converts method calls into attribute-like access, improving object usability and expressiveness.
A method can be called without using parentheses by using @property, which is similar to accessing an attribute. This creates a clearer and easier-to-use interface for object interaction.
Additionally, it offers a deft balance of functionality and encapsulation, protecting object states while delivering an intuitive interface, enabling developers to specify attributes with ease using getter and setter methods.
By combining method functionality with attribute accessibility, the @property
decorator emerges as a crucial tool and offers a straightforward yet effective object interaction paradigm.
An example of Python’s @property
decorator is shown below:
27. How would you create a basic REST API in Python?
In order to build web services that interact via HTTP requests, developers frequently make use of the expressive ability of frameworks like Flask while building a simple REST API in Python.
With its simple and understandable syntax, Flask enables developers to construct routes that can be accessed by a number of HTTP methods, including GET and POST, to communicate with the underlying application.
A REST API built using Flask can easily accept HTTP requests, process the contained data, and provide relevant information in response by specifying unique endpoints linked with various functionality.
In order to ensure seamless communication between various software components in a networked environment, developers can use powerful REST APIs using a combination of Python and Flask.
Here is a little piece of code that uses Flask to create a REST API:
28. Describe how to use the requests library to make an HTTP POST request.
Python’s requests library is a powerful tool that transforms the difficulties of HTTP communication into a welcoming API and makes it simple and natural to interact with online services using HTTP POST requests.
A POST request is made by using the post method, giving the destination URL, and attaching the material to be sent, which can contain form data, JSON, files, and more.
The requests library then manages the underlying HTTP connection, sending the data to the designated URL and collecting the server’s response to enable fluid web interactions.
Developers can easily engage with online services, submit form data, and interface with web APIs through requests, bridging the gap between local apps and the global web.
Using the requests library, the following code sample shows how to send an HTTP POST request:
29. How would you connect to a PostgreSQL database using Python?
Engaging with a PostgreSQL database from a Python environment is handled elegantly by the psycopg2 package, a powerful bridge that allows for seamless database interactions.
By using psycopg2
, programmers can easily create connections, run SQL queries, and get results, directly integrating PostgreSQL’s capability into Python programs.
You can unlock complex database functions with only a few lines of code, guaranteeing that data is accessed, modified, and saved with accuracy and efficiency.
This module allows developers to fully utilize relational databases in their applications by elegantly realizing the synergy between Python and PostgreSQL.
Here is the sample code that demonstrates how to use the psycopg2
library to establish a connection to a PostgreSQL database:
30. What’s the role of ORMs in Python and name a popular one?
Object-relational mapping (ORM) in Python enables developers to connect with databases using Python classes and object paradigms.
It acts as a harmonic mediator between object-oriented programming and relational database administration.
SQLAlchemy, one of the most well-known ORMs in the Python environment, offers a complete set of tools for interacting with multiple SQL databases using high-level, object-oriented syntax.
With the help of SQLAlchemy, database entities can be represented as Python classes, with instances of these classes serving as rows in database tables.
This allows programmers to operate with databases without having to write any raw SQL queries.
Due to the complexity of SQL and database connectivity, ORMs like SQLAlchemy make it possible for more user-friendly, secure, and maintainable database interactions.
Here is a simple example showing how SQLAlchemy works:
31. How would you profile a Python script?
A Python script is profiled by analyzing its computational structure and the time and space details of its execution in order to find any possible performance bottlenecks and improve efficiency.
Developers can carefully analyze the behavior of their code during runtime by utilizing the built-in cProfile
module.
By doing so, they can obtain thorough data on function calls, execution times, and call relationships, allowing them to identify and address performance bottlenecks.
You can guarantee that code not only works correctly but also efficiently, balancing computing resources, and improving overall application performance, by including profiling into the development lifecycle.
Developers can therefore protect programs against inefficiencies by careful profiling, ensuring that they are reliably tuned and performant across a range of computational demands.
Here is a simple example of Python script profiling using the cProfile
module:
32. Explain the GIL (Global Interpreter Lock) in CPython
The Global Interpreter Lock (GIL) in CPython functions as a sentinel, guaranteeing that only one thread runs Python bytecode at a time in a single process, even in multi-threaded applications.
Even though it may appear to be a bottleneck, the GIL is crucial in protecting CPython’s memory management and internal data structures from concurrent access and preserving system integrity.
The need for multithreading in I/O-bound activities, where threads must wait for data to be delivered or received, must be kept in mind, though, since GIL does not eliminate this need.
Thus, even if GIL poses difficulties for CPU-bound activities, comprehension of its behavior and adaptation of techniques, like employing multiprocessing or concurrent programming, allows developers to create effective, concurrent Python programs.
Here is an example of Python code that uses threads and shows how GIL could have an effect on CPU-bound tasks:
33. Explain Python’s async/await. How is it different from traditional threading?
The async/await syntax in Python opens up the world of asynchronous programming, a paradigm that lets some functions cede control to the runtime environment so that other activities can perform in the meanwhile, improving program efficiency.
Async/await maintains activities in a single thread but enables the execution to jump between tasks, assuring non-blocking behavior without the complexity of thread management.
This is in contrast to classical threading, where threads execute in parallel and frequently need complicated management and synchronization.
As a result, developers can handle concurrent I/O-bound activities effectively and with a more straightforward approach to controlling concurrency.
This promotes a cooperative multitasking model in which processes willingly yield control.
As a result, async/await offers a distinctive, simplified way to design concurrent applications, especially where I/O operations are common, finding a balance between performance and complexity.
An example of Python code that uses async/await is provided below:
34. Describe how you would use Python’s concurrent.futures
.
interface for asynchronously executing callables via threads or processes, developers can gracefully manage asynchronous and parallel operations.
This module manages the resource allocation and execution of callables while encapsulating the delicate aspects of threading and multiprocessing through Executors (ThreadPoolExecutor and ProcessPoolExecutor).
Developers can effectively use multi-core processors for CPU-bound activities and provide non-blocking I/O operations by sending tasks to an executor, which can then perform them concurrently and even aggregate their results.
In order to ensure that applications are responsive and performant, concurrent.futures
creates a space where complex calculations and I/O activities can smoothly merge.
Here is a sample of code that uses concurrent.futures
:
35. Compare Django and Flask in terms of use case and scalability.
Two stars in the constellation of Python’s web frameworks, Django and Flask, each shine brightly while meeting various developer requirements.
For programmers creating massive, database-driven applications, Django is the tool of choice since it comes with an ORM and a built-in admin interface.
However, Flask’s simple and modular design gives developers the freedom to select their own components, making it the perfect choice for smaller projects or situations where a lightweight, adaptable solution is essential.
Both frameworks can be scaled to accommodate greater demands when it comes to scalability.
However, Flask’s lean nature allows for customized scaling tactics that are tailored to particular needs, whilst Django’s built-in capabilities can give it a tiny advantage for quick development in bigger, more complicated projects.
Conclusion
Python scripting interviews necessitate an in-depth knowledge of the language’s capabilities, complexities, and applications.
A thorough preparation not only strengthens one’s technical competence but also inspires confidence, helping applicants to move quickly and accurately through the difficult maze of questions.
Aspirants can make sure they are prepared to handle both basic and applied Python problems by reviewing key ideas like concurrency, OOP principles, and data structures, as well as diving into practical applications like web programming and data manipulation.
As a result, having a well-rounded education becomes essential to success and can lead to situations where one’s Python programming abilities can excel and be creative. See Hashdork’s Interview Series for help with interview preparation.
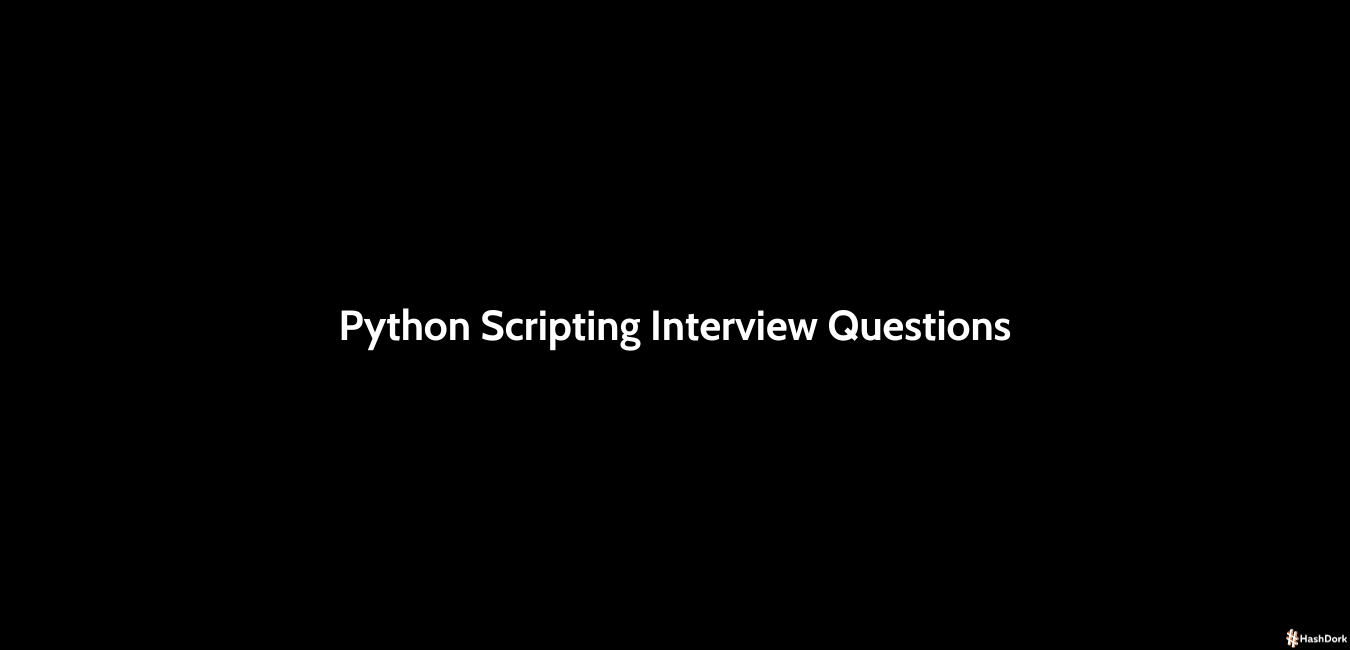
Leave a Reply