Chatbots are very popular these days. So, we have come to help you develop a chatbot using Python. In this post, we will talk about developing an interactive AI chatbot.
Interactive artificial intelligence chatbots are computer systems that replicate human dialogue. Also, they respond to human input using natural language processing and machine learning technologies.
To deliver a more efficient customer care experience, these chatbots may be linked to multiple platforms. Hence, these platforms could be websites, mobile applications, and messaging systems. Besides, they can be used for a variety of purposes, including leisure, education, and advertising.
OpenAI library
The GPT-3 model is available in the OpenAI library. We can use it to produce replies for your chatbot. The package also has a straightforward API for communicating with the model. It is making it simple to integrate into your Python chatbot application.
Hence, you can use OpenAI in your project.
To produce replies from the GPT-3 model, we will use the completion.create() method.
OpenAI also supplies alternative models such as GPT-2, DALL-E, and others. You may use any of these to create your chatbot. However, keep in mind that each model has its unique set of talents, strengths, and shortcomings.
Building the Chatbot
1- First, we must install the OpenAI library and assign the API key received from the OpenAI website. This will provide you access to the GPT-3 model via the OpenAI API.
import openai
openai.api_key = "YOUR_API_KEY"
To set the API key, go to https://beta.openai.com/ and sign up.
2- Now we need to create a chatbot() function that accepts user input. And, it should utilize it as the GPT-3 model’s prompt. The input() method is used to gather the user’s input, and the loop runs until the user inputs “exit”.
def chatbot():
while True:
user_input = input("You: ")
3- If the user input is equivalent to “exit,” the loop will be broken and the chatbot will terminate.
if user_input.lower() == "exit":
break
4- To generate a response from the GPT-3 model, we must now use the openai.Completion.create() function. The engine parameter is set to “text-davinci-002,” which is a GPT-3 model. The prompt parameter is set to the user input, followed by a space to signify the end of the prompt.
The temperature parameter is set to 0.5 to regulate the amount of unpredictability in the generated text. And, the max tokens parameter is set to 2048 to restrict the length of the created answer.
response = openai.Completion.create(
engine="text-davinci-002",
prompt=user_input + " ",
max_tokens=2048,
temperature=0.5
)
5- We will now create a print response from the GPT-3 model.
print("Chatbot: ", response["choices"][0]["text"])
6- We will now add the script’s primary function. When called, it will print the welcome message and then call the chatbot() method.
if __name__ == "__main__":
print("Welcome to the GPT-3 Chatbot!")
print("Type 'exit' to close the chatbot.")
chatbot()
Ask a Different Question to Chatbot
We already talked about the weather. Let’s try something else to improve our conversation. For example, we can ask “How is your mood today?”.
def chatbot():
while True:
user_input = input("You: ")
if user_input.lower() == "exit":
break
elif user_input.lower() == "how is your mood today?":
print("Chatbot: My mood is great, thank you for asking!")
continue
response = openai.Completion.create(
engine="text-davinci-002",
prompt=user_input + " ",
max_tokens=2048,
temperature=0.5
)
print("Chatbot: ", response["choices"][0]["text"])
Other Methods for Developing A ChatBot with Python
Using the Natural Language Toolkit (NLTK) or the SpaCy library
These libraries are great for tasks like tokenization and stemming. Also, they can be used for named entity identification in natural language processing. NLTK is more general-purpose. Also, it offers a broader range of features. However, SpaCy is more performance-focused and is usually thought to be quicker.
You may use the following command to install NLTK:
pip install nltk
To install spacy:
pip install spacy
Using RASA
RASA is an open-source platform for developing conversational AI chatbots. It includes a set of libraries and tools for creating chatbots. Also, it can recognize natural language input and respond appropriately.
You may use the following command to install RASA:
pip install rasa
TensorFlow and Keras
TensorFlow and Keras are prominent machine-learning libraries. You can use it to train a model to recognize natural language input and create suitable answers.
You may run the following command to install TensorFlow:
pip install tensorflow
pip install keras
Conclusion
Interactive artificial intelligence chatbots are computer systems that mimic human communication. Hence, they respond to human input. It is very exciting and promising for the future.
The OpenAI library provides a simple API for connecting with the GPT-3 model. You can design a chatbot that interacts with users naturally and engagingly. You can create a more effective and customized experience, with the correct approach.
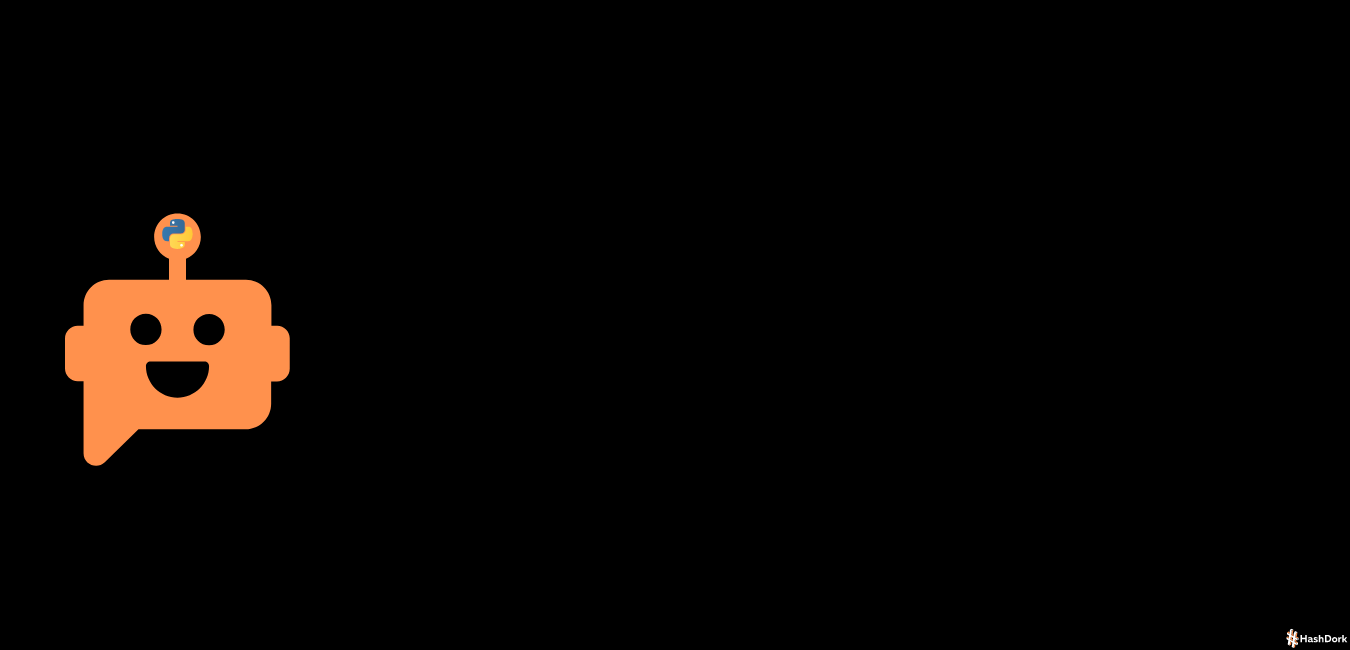
Leave a Reply