Table of Contents[Hide][Show]
- 1. What exactly is TypeScript, and how is it different from JavaScript?
- 2. What are some notable features of TypeScript?
- 3. What are some benefits of using TypeScript?
- 4. What are some drawbacks of using TypeScript?
- 5. What exactly are TypeScript’s components?
- 6. Can you explain interfaces in TypeScript?
- 7. What are variables in TypeScript and how are they declared in various ways?
- 8. How can a subset of an interface be used to generate a new type?
- 9. What do you mean by ‘any’ types, and when should I utilize them?
- 10. How is TypeScript a language with optional statically typed syntax?
- 11. In TypeScript, what do modules mean?
- 12. How do “enums” function in TypeScript?
- 13. What distinguishes an internal module from an external module?
- 14. What does TypeScript’s Anonymous Function mean?
- 15. In TypeScript, what is a namespace and how do you declare one?
- 16. What access modifiers does TypeScript support?
- 17. Does TypeScript allow for function overloading?
- 18. How does TypeScript allow optional arguments in functions?
- 19. How do var, let, and const vary from one another?
- 20. In TypeScript, describe decorators?
- 21. In TypeScript, what do Mixins mean?
- 22. What exactly are Type Aliases in TypeScript?
- 23. What does the term “Scope variable” mean?
- 24. What exactly is noImplicitAny used for?
- 25. How do union and intersection types vary from one another?
- 26. How do you define a TypeScript Declare Keyword?
- 27. In TypeScript, what are generics?
- 28. What does JSX mean in TypeScript?
- 29. What are TypeScript Ambients and when should I utilize them?
- 30. What do you mean by abstract class in TypeScript?
- 31. What exactly is a TypeScript Map file?
- 32. In TypeScript, what are type assertions?
- Conclusion
Microsoft developed and continues to support the TypeScript programming language. With the addition of static typing as an option, it is a syntactical superset of JavaScript.
Large-scale application development language TypeScript compiles JavaScript. Since TypeScript is a superset of JavaScript, programs written in JavaScript are also valid in TypeScript.
Here is a comprehensive list of the most typical TypeScript developer interview questions and responses in case you are attending one.
1. What exactly is TypeScript, and how is it different from JavaScript?
A superset of JavaScript, TypeScript compiles to standard JavaScript. From a conceptual standpoint, TypeScript and JavaScript are similar to SASS and CSS.
In other words, TypeScript is ES6 JavaScript with a few more capabilities. JavaScript is a scripting language more akin to Python, whereas TypeScript is an object-oriented, statically typed language comparable to Java and C#.
Classes and interfaces are all part of TypeScript’s object-oriented design, and type inference is one of the many tools available thanks to its static typing.
JavaScript is written in a file with a .js extension, whereas TypeScript is written in a file with a .ts extension.
Contrary to JavaScript, TypeScript code cannot be directly run in a browser or on any other platform and is not understood by browsers. The target platform will then run the plain JavaScript that was generated from the .ts files using TypeScript’s transpiler.
2. What are some notable features of TypeScript?
- Static typing is a feature of TypeScript that aids type checking during compilation. So, even without executing the script, you can detect mistakes in the code as you write it.
- The DOM can be altered using TypeScript to add or remove items.
- Any Operating System, including Windows, macOS, and Linux, can have the TypeScript compiler installed.
- Classes, interfaces, and modules are some of the functionalities offered by TypeScript. It can thus create object-oriented code for both client-side and server-side development.
- The majority of the features of ECMAScript 2015 (ES 6, 7) are already included in TypeScript, including class, interface, Arrow functions, etc.
- If you’re utilizing JavaScript’s dynamic typing, TypeScript also supports optional static typing.
3. What are some benefits of using TypeScript?
- Any JavaScript engine or browser can execute TypeScript since it is quick, easy to learn, and runs quickly.
- It shares the same syntax and semantics as JavaScript.
- This facilitates the speedier writing of front-end code by backend developers.
- It incorporates ES6 and ES7 capabilities that can function in JavaScript ES5 engines like Node.js.
- Existing JavaScript libraries like Jquery, D3.js, etc. are supported via the Definition file, which has the.d.ts extension.
- A JavaScript script that already exists can invoke the TypeScript code. It also seamlessly integrates with current JavaScript frameworks and libraries.
4. What are some drawbacks of using TypeScript?
- Coding with TypeScript requires a lengthy compilation process.
- It doesn’t support classes that are abstract.
- Type definition files’ quality is an issue.
- Any third-party library must have a definition file in order to be used.
- A compilation step is necessary to convert TypeScript into JavaScript if we want to run the TypeScript application in the browser.
- JavaScript has been used by web developers for many years, and TypeScript doesn’t provide anything new.
5. What exactly are TypeScript’s components?
In TypeScript, there are three main categories of components, including:
- Language: It includes annotations for the type, keywords, and syntax.
- TypeScript Compiler: The TypeScript instructions are translated into JavaScript by this compiler (tsc).
- TypeScript Language Service: The Language Service offers editor-like apps as a second layer on top of the basic compiler process. The standard set of common editing operations is supported by the language service.
6. Can you explain interfaces in TypeScript?
TypeScript uses interfaces to specify the syntax of entities. In other words, data forms like objects or an array of items can be described using interfaces. The interface keyword, the interface name, and its definition are used to declare interfaces. Let’s examine a user object’s basic interface.
The type of a variable can then be set using the interface (similar to how you assign primitive types to a variable). The properties of the interface will then be met by a variable of the User type.
Your TypeScript project benefits from interfaces because they provide uniformity. Additionally, interfaces enhance your project’s tools by enhancing IDE autocomplete capabilities and guaranteeing that the right values are being supplied to constructors and methods.
7. What are variables in TypeScript and how are they declared in various ways?
A variable is a specifically designated area of memory that is used to hold values. A colon (:) is placed after the variable name and is followed by the type when declaring a variable in TypeScript. We declare variables with the var keyword, just as in JavaScript.
Certain guidelines must be observed while declaring a variable in Typescript:
- The name cannot begin with a number.
- The variable name must contain letters or numbers.
- The dollar symbol ($) and underscore (_) are the only special characters that are permitted in this field.
8. How can a subset of an interface be used to generate a new type?
By supplying an existing type or interface and choosing the keys to be excluded from the new type, you can create a new type in TypeScript using the utility type omit.
The example that follows demonstrates how to construct a new type called UserPreview that is based on the User interface but excludes the email property.
9. What do you mean by ‘any’ types, and when should I utilize them?
Sometimes you need to save a value in a variable, but you are unsure of the type of the variable beforehand. The value could originate from user input or an API request, for instance. You can give any type of value to a variable type by using the “any” type.
When a variable’s type is not explicitly specified and the compiler is unable to determine it from the context, TypeScript believes the variable is of type any.
10. How is TypeScript a language with optional statically typed syntax?
It is possible to instruct the compiler to disregard a variable’s type in TypeScript since it is optionally statically typed. Any data type can be used to assign any kind of value to the variable. When being compiled, TypeScript won’t provide any error checking.
11. In TypeScript, what do modules mean?
It is effective to group together relevant variables, functions, classes, interfaces, etc. using modules. It can be used, but not in the global scope, only inside its own scope.
In essence, a module’s defined variables, functions, classes, and interfaces cannot be directly accessed from outside the module.
The export keyword can be used to build a module, while the import keyword can be used to include a module in another module.
12. How do “enums” function in TypeScript?
A method of defining a collection of named constants is to use enums or enumerated types. These data structures have a fixed length and a collection of fixed values.
When representing a collection of alternatives for a given value in TypeScript, enums are frequently used to describe the possibilities using a set of key/value pairs.
Let’s see an illustration of an enum used to provide a variety of user kinds.
Enums are internally converted into regular JavaScript objects by TypeScript following compilation. The usage of enums is therefore preferable to the use of several separate const variables.
Your code is type-safe and easier to comprehend because of the grouping that enums provide.
13. What distinguishes an internal module from an external module?
Internal Module:
- Internal modules were a feature of Typescript’s previous iteration.
- The name and body of internal modules are defined using ModuleDeclarations.
- These are members of other modules that are local or exported.
- Classes, interfaces, functions, and variables are grouped together in internal modules, which can be exported into another module.
External Module:
- In the most recent version, external modules are referred to as modules.
- A separate source file known as an external module is used to create them, and each one has at least one import or export declaration.
- In the most recent version, external modules are referred to as modules.
- The internal statements of the module definitions can be hidden using external modules, leaving just the methods and arguments connected to the defined variable visible.
14. What does TypeScript’s Anonymous Function mean?
Functions classified as anonymous lack a function name as an identifier. These routines are dynamically defined during runtime. Like regular functions, anonymous functions can take inputs and produce results.
An anonymous function is typically unreachable once it has been created. Variables can be assigned to an anonymous function.
15. In TypeScript, what is a namespace and how do you declare one?
Namespace logically groups functionalities. These internally preserve the typescript legacy code. It encompasses the traits and things with particular connections.
Another name for a namespace is an internal module. Interfaces, classes, functions, and variables can all be included in a namespace to provide a collection of connected functionality.
16. What access modifiers does TypeScript support?
The following are examples of how the public, private, and protected access modifiers in TypeScript can be used to control a class member’s accessibility:
- Public – Access is available to every member of the class, all of its descendant classes, and every instance of the class.
- Protected – They are accessible to every member of the class and all of its subclasses. However, a class instance cannot access.
- Private-Access to them is restricted to class members only.
Since JavaScript is handy, if an access modifier is not given, it is assumed to be public.
17. Does TypeScript allow for function overloading?
Yes, function overloading is supported by TypeScript. But now it’s being done is strange. Therefore, when overloading with TypeScript, there is just one implementation with a variety of signatures.
While the second signature contains a parameter of type text, the first signature only has one parameter of type number. The third function, which includes an argument of type any, contains the actual implementation.
The implementation then determines the type of the provided parameter and runs a separate section of code in accordance with that determination.
18. How does TypeScript allow optional arguments in functions?
Contrary to JavaScript, if you attempt to call a function without specifying the precise amount and kinds of parameters as stated in its function signature, the TypeScript compiler will raise an error.
You can utilize optional parameters by utilizing the question mark symbol (‘?’) to get around this issue. It shows that optional parameters can be denoted by adding a “?” to those that may or may not receive a value.
19. How do var, let, and const vary from one another?
Three different ways to declare a variable are offered by TypeScript, each with a suggested application.
var: Declares a global or function-scoped variable with JavaScript’s var variables’ behavior and scoping guidelines. Variables do not need their values to be set when they are declared.
let: declares a locally scoped block variable. Let variables don’t need a variable’s value to be set when they are declared. The term “block-scoped local variable” refers to a variable that can only be accessed from inside the block in which it is contained, such as a function, an if/else block, or a loop. Let variables cannot be read from or written to before they are declared, in contrast to var.
const: Declares a value for a block-scoped constant that cannot be altered after it has been initialized. Const variables need to be initialized before being declared. For variables that remain constant during their entire existence, this is optimal.
20. In TypeScript, describe decorators?
An accessor, property, parameter, class, function, or decorator can all be declared in a specific way as decorators. Decorators are functions with the @expression prefix that will be invoked at runtime with details about the decorated declaration.
The expression must evaluate a function in order for decorators to work.
TypeScript Decorators provide the goal of declaratively adding annotations and information to the current code.
In our tsconfig.json file or on the command line, you must activate the experimentalDecorators compiler option in order to enable experimental support for decorators:
21. In TypeScript, what do Mixins mean?
Mixins are a technique of creating classes from reusable parts in Javascript and mixing partial classes to create more complex full classes.
The concept is straightforward: function B accepts class A and returns a new class with its functionality added, as opposed to class A extending class B to obtain its functionality. Function B in this case is a mixin.
22. What exactly are Type Aliases in TypeScript?
Type aliases change the name of a type. Type aliases, like interfaces, can be used to name primitives, unions, tuples, and any other types that would otherwise have to be defined by hand.
Aliasing does not create a new type; rather, it changes the name of an existing type. Aliasing a primitive isn’t really practical, although it can be beneficial for documentation.
Type aliases, like interfaces, can be universal; all you have to do is add type parameters and use them on the alias declaration’s right side.
23. What does the term “Scope variable” mean?
JavaScript supports both local and global scope variables. The scope is a collection of objects, variables, and functions.
An example of declaring a variable in two scopes is:
- Variable with Local Scope – It serves as a function object for usage within functions.
- Variable with global scope – This window object can be used both within and outside of functions
24. What exactly is noImplicitAny used for?
The TypeScript compiler’s treatment of your project’s implicit any types is altered by the noImplicitAny property in the tsconfig.json configuration file for TypeScript projects.
The noImplicitAny flag can be set to true or false and is always changeable after initialization. Given that every project is unique, there is no right or wrong answer on what this number should be.
Understanding the distinctions between the flag’s on and off states can help you select what setting to use for the flag.
The compiler does not determine the type of a variable based on how it is used if the noImplicitAny flag is set to false (the default). The type is instead set to any by default by the compiler.
However, if the noImplicitAny option is set to true, the compiler will make an effort to infer the type and will raise a compile-time error if it is unable to do so.
25. How do union and intersection types vary from one another?
Instead of generating new kinds from scratch, unions and intersection types allow you to compose and mix existing types. Both union and intersection have distinctive qualities that make them perfect for certain use cases.
A type that can be one of several kinds is referred to as a union type. The list of types that will be utilized in the new type is divided using the | (vertical bar) symbol in union types.
Let’s look at an illustration:
On the other hand, the intersection is defined as a type that merges many types into one, integrating all of each type’s features to form a new type. The list of kinds that will be merged is divided into intersections using the & symbol.
Let’s examine an illustration:
26. How do you define a TypeScript Declare Keyword?
There are no TypeScript declaration files in JavaScript libraries or frameworks. However, you must utilize the declare keyword in order to use them in the TypeScript file without encountering any compilation errors.
When you wish to specify a variable that could already exist somewhere else, you use the declare keyword in ambient declarations and methods.
27. In TypeScript, what are generics?
A tool called TypeScript Generics offers a method for producing reusable parts. Instead of working with only one form of data, it can develop components that can operate with a range of data types.
Additionally, it offers type safety without sacrificing efficiency or productivity. We can build generic classes, generic functions, generic methods, and generic interfaces thanks to generics.
Generics are highly typed collections since a type parameter is written in between open () and close (>) brackets. It makes use of a unique sort of type variable called T which stands for types.
28. What does JSX mean in TypeScript?
JSX is a legitimate JavaScript that can be converted from an embeddable XML-like syntax. Along with the React framework, JSX gained popularity.
The direct compilation, type verification, and embedding of JSX into JavaScript are supported by TypeScript. You must give your file a.tsx extension and activate the JSX option if you wish to utilize it.
29. What are TypeScript Ambients and when should I utilize them?
The compiler is informed about the real source code that is located elsewhere through ambient declarations.
It will break without any prior notice if we attempt to utilize these source codes at runtime but they are not present. Document-like files are ambient declarations files.
If the source changes, the documentation must be updated, and compiler issues will result if the ambient declaration file is not changed.
Additionally, it enables us to leverage well-known JavaScript libraries that are already widely used, such as jQuery, AngularJS, NodeJS, etc.
30. What do you mean by abstract class in TypeScript?
Abstract classes define an object’s contract without allowing for direct object instantiation. However, an abstract class could also offer its member’s implementation information.
One or more abstract members can be found in an abstract class. The abstract members of the superclass must then have an implementation provided by any classes that extend the abstract class.
Let’s take a look at a TypeScript example of an abstract class and how a different class might extend it. Although both the Car and the Bike classes in the example below extend the Vehicle class, they each implement the drive() function in a unique way.
31. What exactly is a TypeScript Map file?
A source map file called a TypeScript Map file contains data about our original files. The source map files, or .map files, allow tools to map between the JavaScript code that is output and the TypeScript source files that produced it.
Additionally, these files can be consumed by debuggers, allowing us to debug the TypeScript file rather than the JavaScript file.
32. In TypeScript, what are type assertions?
While type assertion functions similarly to typecasting in other languages like C# and Java, it doesn’t carry out type verification or data rearrangement.
While type assertion has no effect on runtime, type casting offers this support. Type assertions, on the other hand, are only utilized by the compiler and provide guidance on how we want our code to be examined.
Conclusion
In conclusion, we hope these TypeScript interview questions will be helpful to you, whether you’re a developer getting ready for a job interview or a hiring manager trying to find the best applicant.
Remember that the recruiting process involves more than just technical expertise. To ensure that you land (or locate the suitable applicant for) the job, past experiences and soft skills are equally crucial.
A lot of TypeScript interview questions are open-ended and don’t have a single right answer, so keep that in mind.
Interviewers are interested in the rationale behind your responses.
Always be ready for follow-up inquiries explaining how you arrived at your answer. Describe your thought process.
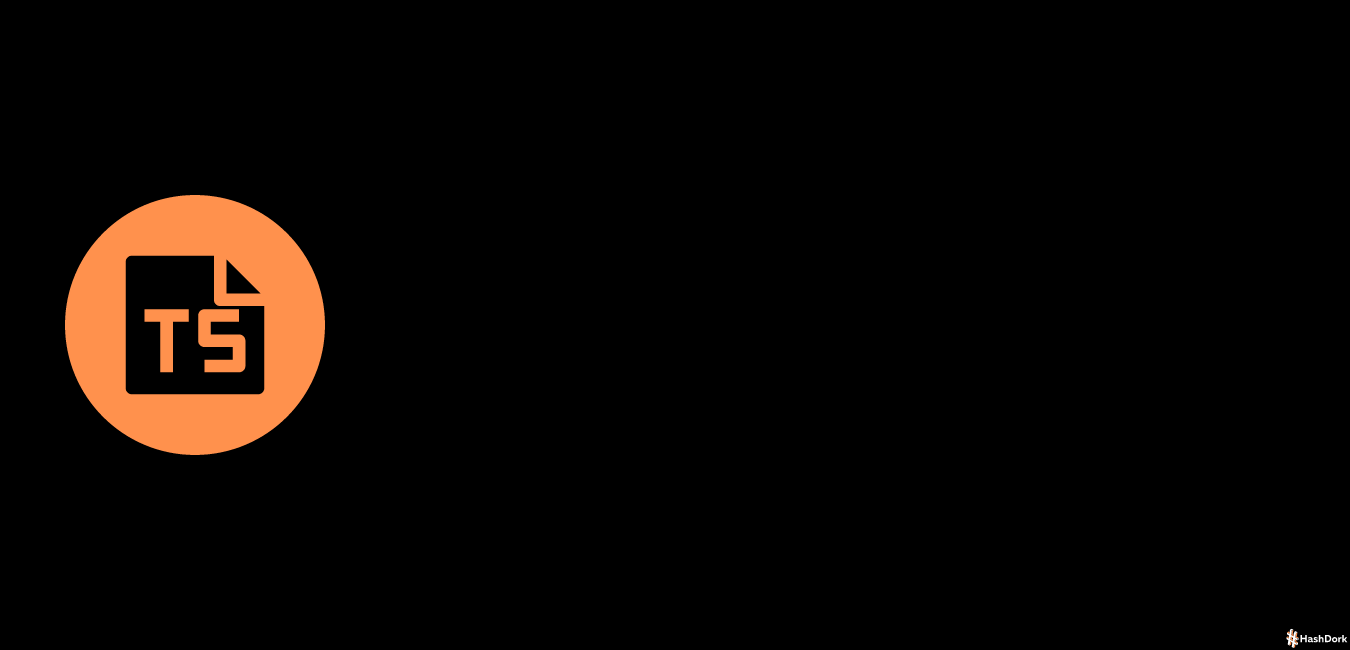
Leave a Reply